This is the second exercise in the series. You may want to start with the first one.
React is a JavaScript library for building dynamic websites. It works by reacting to changes in the data, and making changes in what the user sees based on those changes. The data it reacts to are called props and state.
Props – Input data to the component
State – Internal state data of the component
Set Up
If you haven’t already, you’ll need to get the exercise files. If you already got them when you did the first exercise, you don’t need to get them again.
To get them, clone this repository to your computer:
https://github.com/natafaye/props-and-state-practice
You can clone it using git with this command:
git clone https://github.com/natafaye/props-and-state-practice
Open the props-and-state-practice folder in Visual Studio Code.
Open a terminal in this folder (Ctrl + `
or Terminal > New Terminal)
Install the dependencies that are in the package.json file, with this command:
npm install
Exercise 2: Class Components
Expand the src folder, then the exercise-2-class folder.
In this folder there are three class components, the App component, the Contact component, and the ContactList component. You can mix functional and class components, but for this exercise we’ll use all class components to practice with them.
There is also a data.js file that holds some static data that we will use. In a real app, we might pull our data from a server, but for this exercise we’ll just pull it from the data.js file.
Set Up
We need to make sure our index.js is using the App component in this folder. Open index.js in the src folder, and make sure that the import for Exercise 2: Class Component is uncommented:
// Exercise 2: Class Components
import App from './exercise-2-class/App';
All the other App imports should be commented out.
The Goal
In Exercise 1 we set up our Contact component to show data about a contact.
Now we want our app to actually maintain some internal state data (a list of contacts) and show that data using our Contact List component, which uses the Contact component.
Our App should react to any changes in the list of contacts and re-render, which will re-render the ContactList component and the Contact components inside it.
The list of contacts should start out set to the array in the variable INITIAL_CONTACTS
in the data.js file.
So, when it’s all completed, our app should look like this:
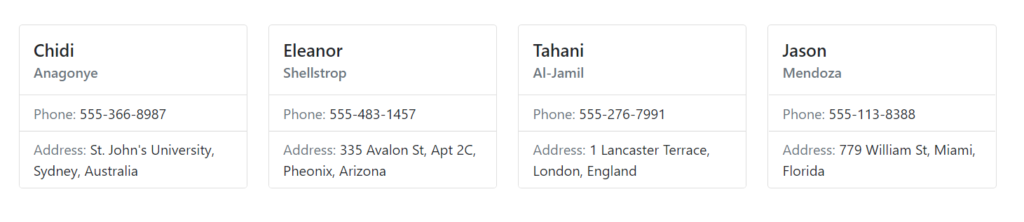
Tips
The Contact component is identical to the one in Exercise 1. You can copy your code from Contact.js in the exercise-1-class folder, into Contact.js in the exercise-2-class folder.
We will store our contacts in the state of the App component. Our ContactList and Contact components are purely presentational. Presentational means they only know how to present the data, they don’t manage the data or have any data in state. They just react to changes in their props, which will flow down to ContactList from App (and to Contact from ContactList).
React needs to be able to tell exactly what changed when it rerenders your component. Any component that displays a list needs to put a key attribute on the top JSX element (component or HTML element) of each item. The key should be set to something that is unique to each item in the list. It’s most often an ID.
Hints
How do I store the contacts in the state of the App component?
How do I access the list of contacts I stored in the state of the App component?
How do I get the list of contacts from the App component to my ContactList component?
How do I use the list of contacts in the ContactList component?
Okay, I have the list of contacts. How do I use that list to show each contact with the Contact component?
How many props & state variables should I use here?
I need more help. What might it look like to save the list of contacts in the state of the App component?
I need more help. What might it look like to get the list of contacts to the ContactList component?
I need more help. How do I set up the ContactList component to use the Contact component?
Exercise 2: Functional Components
Expand the src folder, then the exercise-2-functional folder.
In this folder there are three functional components, the App component, the Contact component, and the ContactList component. You can mix functional and class components, but for this exercise we’ll use all functional components to practice with them.
There is also a data.js file that holds some static data that we will use. In a real app, we might pull our data from a server, but for this exercise we’ll just pull it from the data.js file.
Set Up
We need to make sure our index.js is using the App component in this folder. Open index.js in the src folder, and make sure that the import for Exercise 2: Functional Component is uncommented:
// Exercise 2: Functional Components
import App from './exercise-2-functional/App';
All the other App imports should be commented out.
The Goal
In Exercise 1 we set up our Contact component to show data about a contact.
Now we want our app to actually maintain some internal state data (a list of contacts) and show that data using our Contact List component, which uses the Contact component.
Our App should react to any changes in the list of contacts and re-render, which will re-render the ContactList component and the Contact components inside it.
The list of contacts should start out set to the array in the variable INITIAL_CONTACTS
in the data.js file.
So, when it’s all completed, our app should look like this:
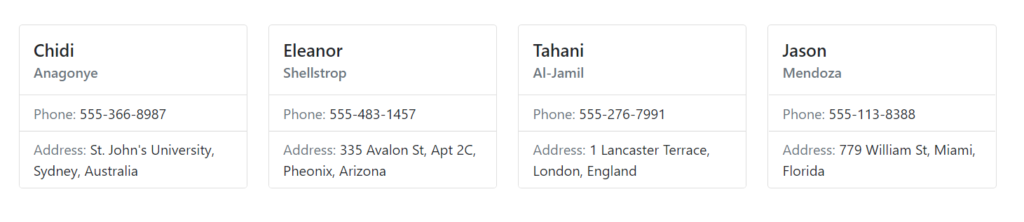
Tips
The Contact component is identical to the one in Exercise 1. You can copy your code from Contact.js in the exercise-1-functional folder, into Contact.js in the exercise-2-functional folder.
We will store our contacts in the state of the App component. Our ContactList and Contact components are purely presentational. Presentational means they only know how to present the data, they don’t manage the data or have any data in state. They just react to changes in their props, which will flow down to ContactList from App (and to Contact from ContactList).
Hints
How do I store the contacts in the state of the App component?
How do I access the list of contacts I stored in the state of the App component?
How do I get the list of contacts from the App component to my ContactList component?
How do I use the list of contacts in the ContactList component?
Okay, I have the list of contacts. How do I use that list to show each contact with the Contact component?
How many props & state variables should I use here?
I need more help. What might it look like to save the list of contacts in the state of the App component?
I need more help. What might it look like to get the list of contacts to the ContactList component?
I need more help. How do I set up the ContactList component to use the Contact component?
Conclusion
Were you able to get all the exercises working? Do you understand how to use props and state a little better?