Let’s practice with Javascript for and for-of loops.
Review
Remember, a for loop in Javascript works like this:
And a for-of loop in Javascript works like this:
The Challenge
Your goal is to write a Javascript function that will fill up a shopping basket with the correct items based on three variables:
- numFish – This is a global variable that holds how many fish to put in the basket, for example: 3
- numLemons – This is a global variable that holds how many lemons to put in the basket, for example: 0
- otherItems – This is a global variable that holds an array of the other items to put in the basket (one of each), for example: [“hamburger”, “cookie”, “egg”]
You don’t need to worry about setting these variables, another function handles that when the Get New Shopping List button is clicked.
What you’ll need to do is write three loops – two for loops and one for-of loop – inside the fillBasket() function.
- The first loop will add the fish to the basket
- The second loop will add the lemons to the basket
- The third loop will add the other random items to the basket
To add something to the basket, call the addItemToBasket() function (this is a pre-written function) and pass in the name of the item as a parameter. So this would add a lemon to the basket:
addItemToBasket("lemon");
You’ll also want to make sure you’re calling the emptyBasket() function (another pre-written function) at the beginning of your fillBasket() function so you start out with an empty basket.
Testing Your Code
You’ll be able to tell that your loops are working when you see the correct icons in the shopping basket.
For example, if you clicked the Get Shopping List button and got this:
- 6 fish
- 1 lemon
- hamburger
- cookie
- bacon
- bone
When you click the Fill Basket button (which runs the fillBasket() function), your basket should look like this:
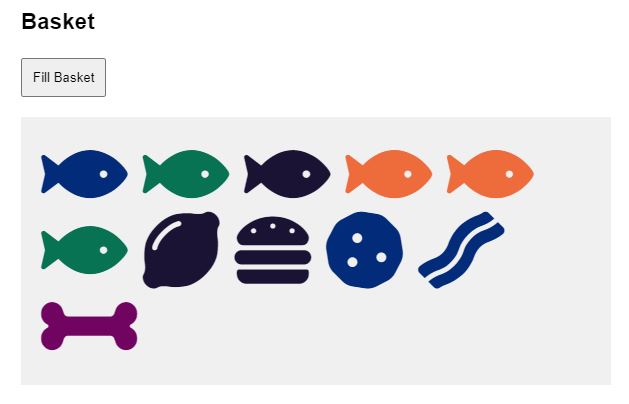
Get Started
Click the Edit on Codepen button and fork this Codepen to get started!
See the Pen Stretch Challenge: For Loops by Natalie Childs (@natafaye) on CodePen.light
Good luck!
Got Stuck?
If you’ve gotten stuck, see this post for hints:
Inner Workings
The inner workings for this challenge – including the code for the emptyBasket() and the addItemToBasket() functions – are in this Codepen:
See the Pen Stretch Challenge: For Loops – Inner Workings by Natalie Childs (@natafaye) on CodePen.light