There are many different ways to use Git & GitHub in a project, depending on the needs and structure of the project. And Git has a lot of advanced features that are valuable and important when you become a more advanced programmer. But when you’re starting out, it can all get a bit overwhelming. Here’s a cheat sheet for using Git with GitHub in simple, usable ways for beginners.
Setting Up
- You have a folder of files on your computer that you want in a repository on GitHub
- You want to create a local repository on your computer
- You have a local repository and you want to push your files to a remote repository on GitHub
- You need the URL for your remote repository
- You want to create a remote repository on GitHub
- There’s a remote repository that you want on your computer
Making Changes
- You’ve made changes on your computer and you want to push those changes to the remote repository on GitHub
- You’ve got a merge conflict
- You want to check the status of your local repository
- You pushed your changes to a remote repository, but you’re not seeing them on GitHub
- You want to change which remote repository your local repository is connected to
- Your local React repository won’t let you pull or push to your remote GitHub repository
You have a folder of files on your computer that you want in a repository on GitHub:
First you’ll need to create a local repository in that folder:
You want to create a local repository on your computer.
Next you’ll create a remote repository on GitHub:
You want to create a remote repository on GitHub
Then you’ll push your local repository to the remote repository on GitHub:
You have a local repository and you want to push your files to a remote repository on GitHub.
You want to create a local repository on your computer:
Rut this command in the folder you want to become a local repository:
git init
You have a local repository and you want to push your files to a remote repository on GitHub:
git remote add origin <remote-repository-url-goes-here>
git branch -M main
git push -u origin main
To get your remote repository URL:
You need the URL for your remote repository.
You need the URL for your remote repository:
To get your repository URL, go to your repository on GitHub.
You may see a Code button. Click the Code button and copy the HTTPS URL.
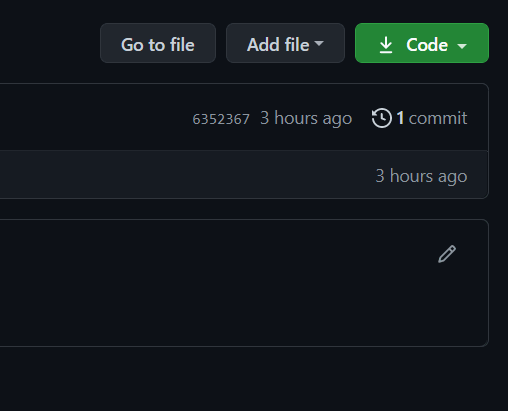
Or you may see a Quick Setup section where you can copy the HTTPS URL.

In this example, if I wanted to connect my local repository to the remote repository in the picture above, I would run:
git remote add origin https://github.com/natafaye/verbose-adventure
If you don’t have a remote repository, you’ll want to create one:
You want to create a remote repository on Github
You want to create a remote repository on GitHub:
Log in to GitHub, go to the top right corner, and click the + button. Then select New repository.
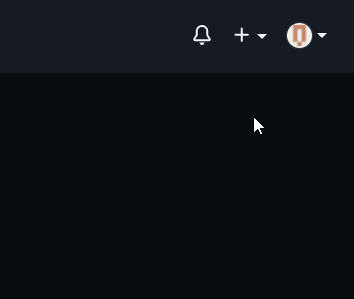
Give your repository a name, select Public or Private, and click the Create repository button.
There’s a remote repository that you want on your computer:
git clone <remote-repository-url-goes-here>
To get the remote repository URL:
You need the URL for your remote repository
If the repository has a package.json
file, you may need to install the node modules because they are typically not committed to the repository. You can do that with this command:
npm install
You’ve made changes on your computer and you want to push those changes to the remote repository on GitHub:
git add .
git commit -m "A description of your changes"
git pull
git push
When you run git pull you may get this error:
There is no tracking information for the current branch.
Please specify which branch you want to merge with.
See git-pull(1) for details.
git pull <remote> <branch>
If you wish to set tracking information for this branch you can do so with:
git branch --set-upstream-to=origin/<branch> main
This happens when your local repository isn’t sure which remote repository and branch you want to pull from. You can fix that by specifying the remote repository and the branch, like this:
git pull origin main
You can avoid this problem in the future by telling your local repository which remote repository (or upstream) you want it connected to. Next time you push, do it like this:
git push -u origin main
You’ll get the message:
Everything up-to-date
Branch 'main' set up to track remote branch 'main' from 'origin'.
This means your local repository is connected to your remote repository and now you can use git pull
and git push
without problems.
You’ve got a merge conflict:
You may get a merge conflict when you run git pull
or merge in a branch if someone else has made changes to the same lines you have. To resolve the merge conflict, open the file or files that have conflicts, and change those files to look like you want them to.
You can use the Visual Studio Code helper buttons:
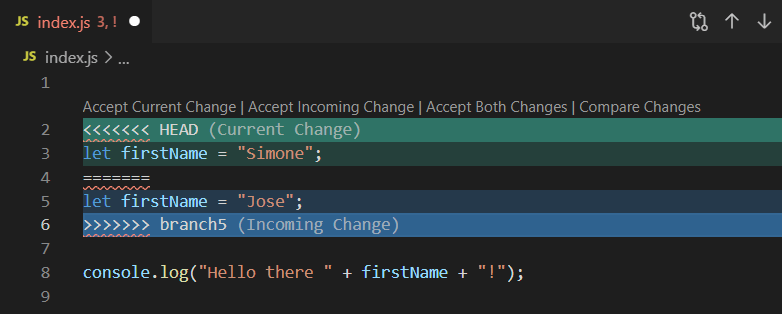
Or just edit the file directly:
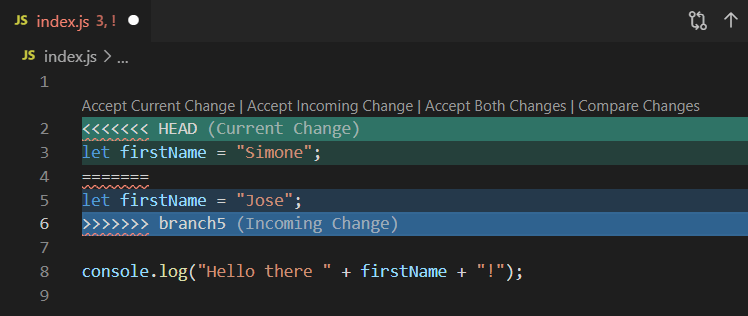
Then add, commit, pull and push:
git add .
git commit -m "Fixed merge conflict"
git pull
git push
You want to check the status of your local repository
git status
There are a few possible responses you might get.
Working Tree Clean
On branch main
Your branch is up to date with 'origin/main'.
nothing to commit, working tree clean
This means any changes you’ve made have been added and committed (or that you’ve made no changes). You are ready to push your changes to the remote repository.
Changes not staged for commit
On branch main
Your branch is up to date with 'origin/main'.
Changes not staged for commit:
(use "git add <file>..." to update what will be committed)
(use "git restore <file>..." to discard changes in working directory)
modified: README.md
no changes added to commit (use "git add" and/or "git commit -a")
This means your changes have not been added. You’ll need to add them (and then commit them)
git add .
git commit -m "Description of your changes"
Changes to be committed
On branch main
Your branch is up to date with 'origin/main'.
Changes to be committed:
(use "git restore --staged <file>..." to unstage)
modified: README.md
This means your changes have been added, but have not been committed. You’ll need to commit them.
git commit -m "Description of your changes"
You pushed your changes to a remote repository, but you’re not seeing them on GitHub
You may have forgotten to save your changes. Git can only push saved changes.
You may have forgotten to add and/or commit your changes. Check the status of your local repository
You want to check the status of your local repository
You may have pushed your changes to the wrong remote repository. Check which repository you’re pushing to with
git remote -v
This will tell you where your local repository is set up to push to and fetch (or pull) from. If the repository URLs for origin are not set correctly, changing those should fix things:
You want to change which remote repository your local repository is connected to
You want to change which remote repository your local repository is connected to:
git remote set-url origin <remote-repository-url-goes-here>
To get your remote repository URL:
You need the URL for your remote repository.
Your local React repository won’t let you pull or push to your remote GitHub repository
This can happen if you created your GitHub repository with a README file. When you try to push
you get an error like this:
To https://github.com/natafaye/verbose-adventure
! [rejected] main -> main (non-fast-forward)
error: failed to push some refs to 'https://github.com/natafaye/verbose-adventure.git'
hint: Updates were rejected because the tip of your current branch is behind
hint: its remote counterpart. Integrate the remote changes (e.g.
hint: 'git pull ...') before pushing again.
hint: See the 'Note about fast-forwards' in 'git push --help' for details.
But when you try to pull
you get something like this:
From https://github.com/natafaye/verbose-adventure
* branch main -> FETCH_HEAD
fatal: refusing to merge unrelated histories
The problem is that you have two entirely different commit histories on each of your repositories. You can think of it like this:
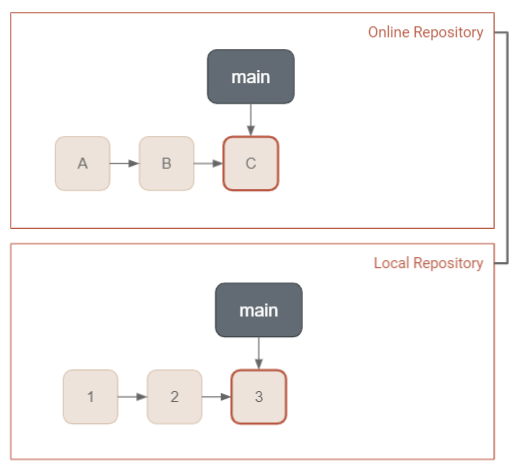
The online repository has a commit history (from adding the README) and the local repository has a commit history (from create-react-app). But these two histories are completely unrelated. Git gets mad and “refuses to merge unrelated histories.”
To fix this, we can just force Git to merge them. As long as it’s just the README in the online repository that’s causing the problem, it shouldn’t create issues to force them to merge.
We can do that with:
git pull origin master --allow-unrelated-histories
It’s likely you’ll get a merge conflict you’ll need to resolve:
You’ve got a merge conflict
Once you’ve resolved that merge conflict and added and committed the changes, you should be able to pull from and push to your online repository.